Review on Website- Custom Integration
To enable this, go to the Review on Website activity in Program Settings >> Activities. Select "Custom" in the Review Provider dropdown.
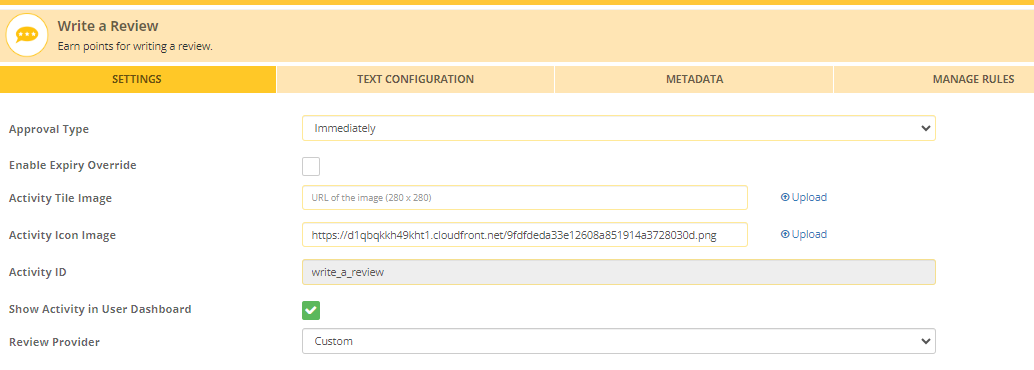
There are two ways to award loyalty points for this custom activity.
API
You can award points using the Award API endpoint.
Here are a few code snippet examples to help you with the implementation.
import requests
import json
headers = {'partner-id': 'your_partner_id',
'api-key': 'your-api-key'}
payload = {"member_id": "[email protected]",
"points_passed": 100,
"activity_id": "write_a_review"}
response = requests.post(url = "https://api.zinrelo.com/v2/loyalty/transactions/award",
headers = headers,
data = payload)
if response.ok:
info = json.loads(resp.content)
if info:
#Call a function to notify the user
$fields = array('activity_id' => 'write_a_review',
'points_passed'=>100,
'member_id' => '[email protected]');
$fields_string = '';
foreach($fields as $key=>$value){
$fields_string .= $key.'='.$value.'&';
}
rtrim($fields_string, '&');
$curlhandle = curl_init();
curl_setopt($curlhandle, CURLOPT_URL, 'https://api.zinrelo.com/v2/loyalty/transactions/award');
curl_setopt($curlhandle, CURLOPT_POST, true);
curl_setopt($curlhandle, CURLOPT_POSTFIELDS, $fields_string);
curl_setopt($curlhandle, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curlhandle, CURLOPT_SSL_VERIFYPEER, 0);
$headers = array();
$headers[] = 'partner-id:your_partner_id';
$headers[] = 'api-key: your-api-key';
curl_setopt($curlhandle, CURLOPT_HTTPHEADER, $headers);
$contents = curl_exec ($curlhandle);
$httpcode = curl_getinfo($curlhandle, CURLINFO_HTTP_CODE);
if ($httpcode==200){
$info = json_decode($contents);
if ($info){
//Call a function to notify user
}
}
curl_close ($curlhandle);
require "httparty"
response = HTTParty.post('https://api.zinrelo.com/v2/loyalty/transactions/award',
:query=>{:member_id=>"[email protected]",
:points_passed=>100,
:activity_id=>'write_a_review'},
:headers=>{"partner-id" => "your_partner_id",
"api-key" => "your-api-key"})
if response.code == 200
body = response.to_s
if body
#Call a function to notify the user
Batch Mode
You can also award points for this activity using our batch mode processing technique.
Batch mode allows you to handle any custom activity performed by your users. This requires you to upload the user activity files in a specified format at a pre-specified FTP location. These files will then be read and processed by Zinrelo on a daily basis. To know more about batch mode processing technique, click here.
End User Experience
- The member will log in to the website store and navigate to the dashboard.
- After successfully logging in, the member will locate and click on the "Earn Points" tab.
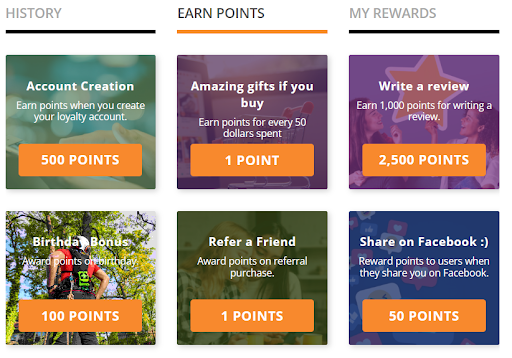
- Subsequently, the member will choose the "Write a Review" activity from the available options.
- To proceed with submitting a review, the member needs to click on a hyperlink text or button.
- At this point, the member may be prompted to provide their email address or create a new account if necessary.
- Once the necessary information is provided, the page for submitting the review will open up.
- After the review has been submitted, it will go through a review process conducted by a reviewer.
- Upon approval of the submitted review by the reviewer, the member will be rewarded with the corresponding points.
What is the process of awarding the points for "Review on website" activity?
The process of awarding points for the "Review on Website" activity typically involves the following steps:
- A member posts a review or comments on a specific product, service, or piece of content.
- The review goes through a moderation process where a designated reviewer or moderator evaluates the review. The reviewer verifies the authenticity and appropriateness of the review.
- Once the reviewer approves the review, the trigger to award points is made to Zinrelo.
- The system calculates the appropriate number of points based on predefined rules and configurations set for the activity.
- The points are awarded to the member for their review submission.
Updated 11 months ago