Setting up in-cart redemption with Zinrelo
Enabling members to redeem their rewards in their shopping cart without leaving the purchase flow achieves multiple objectives.
- Makes redemption easy for users
- Increases conversion percentage
- Users get hooked to the loyalty rewards program and come back for more purchases
Introduction
In-cart redemption of rewards is implemented on the payment page in the checkout process. The implementation of in-cart redemption can be done via the JavaScript and API. It involves 4 simple steps:
- Fetch Eligible Rewards- You can fetch the eligible rewards via JavaScript and server-side API. This will return an array of eligible rewards.
- Display Eligible Rewards- All eligible rewards of the member will be displayed.
- Apply the reward to the cart- Member chooses a reward and clicks on the Apply button. The reward will be applied to the users’ cart.
- Confirm the Redemption- Once the purchase is completed, call Zinrelo's API to confirm the redemption and deduct the points from the member's loyalty account.
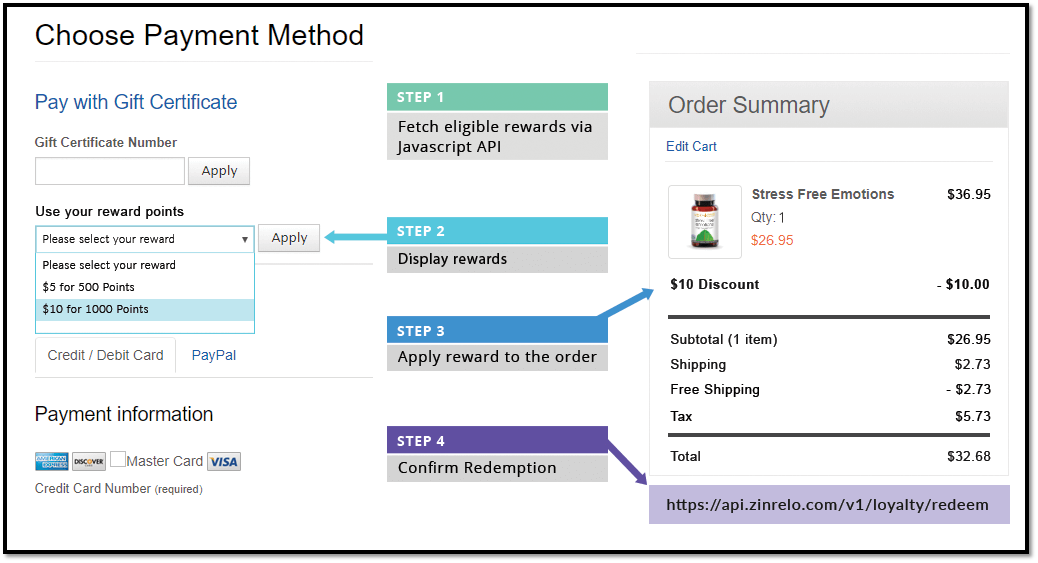
Note: In some cases, coupon codes are required. In those cases, either you can generate the coupon codes dynamically or call our API to display all system-stored coupon codes. The user will apply the reward and the purchase will happen.
If the member removes the coupon code, the points that were deducted are returned to the member’s account and will reflect on the cart page. The coupon code will be invalid then and can never be used again.
The steps of in-cart redemption of rewards are detailed below:
Step 1: Fetch Eligible Rewards
You can fetch eligible rewards via JavaScript and through our server-side Get Eligible Rewards API. The JavaScript code is mentioned below:
//----
get_zrl_rewards = function() {
//Initialize the code and check whether the Zinrelo all.js file has loaded
//If the Zinrelo all.js file has loaded successfully, fetch user rewards
if (typeof(zrl_mi) !== 'undefined' &&
zrl_mi.init_success !== 'undefined' &&
zrl_mi.init_success) {
get_user_rewards();
} else {
//Continue in a recursive loop to check for all.js file initialization
setTimeout(get_zrl_rewards, 500);
}
}
//----
//----
//Function to fetch rewards that this user is eligible for
get_user_rewards = function() {
//If the user email field is not present or is blank, then exit
if (!zrl_mi.user_email || zrl_mi.email === '') {
return false;
}
//Otherwise, make an Ajax call to fetch the list of eligible rewards
var data;
data = {
merchant_id: zrl_mi.partner_id,
user_email: zrl_mi.user_email
};
return ss_mi.ajax({
url: "" + zrl_mi.server + "/user/rewards",
data: data,
//If Ajax call successful, display the rewards
success: show_rewards,
//If Ajax call fails, handle this error
onerror: handle_error
});
};
//----
//Call to the main function to fetch eligible rewards on the webpage
get_zrl_rewards();
Note: The abovementioned code will return only rewards which have been configured for in-cart redemption in the Zinrelo Admin Console.
The sample code for show_rewards and handle_error function is further described in Step 2 below.
Shown below is a sample response that you will get from the JavaScript Ajax call. This response contains an array with a list of rewards for which the member is eligible. This response is automatically populated in the array zrl_mi.user_rewards when the Ajax call returns successfully. You can use this array to construct your rewards display on the shopping cart webpage.
[{
"giftcard_description": "$5 OFF for 500 points",
"redemption_name": "$5 discount",
"redemption_type": "Fixed Amount Discount",
"allowed_redeem_points": 1000,
"redemption_id": "reward_3063f",
"redemption_value": 10
},
{
"giftcard_description": "$10 OFF for 1000 points",
"redemption_name": "$10 discount",
"redemption_type": "Fixed Amount Discount",
"allowed_redeem_points": 2000,
"redemption_id": "reward_16383",
"redemption_value": 20
},
{
"giftcard_description": "Free Shipping up to $50",
"redemption_name": "Free Shipping",
"redemption_type": "Free Shipping",
"allowed_redeem_points": 0,
"redemption_id": "reward_15323",
"redemption_value": ""
}]
Step 2: Display Eligible Rewards
This step defines the success and the error functions used in Step 1. Within the success function, the response in Step 1 is used to display the eligible rewards to the member.
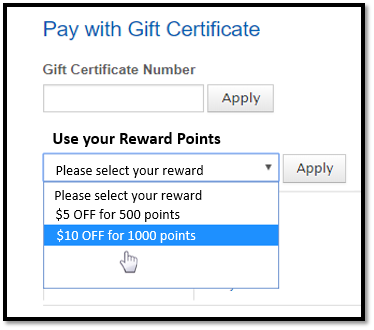
Success Function
The sample code for the success function show_rewards used in Step 1 is defined here. This function takes the data array zrl_mi.user_rewards from the response in Step 1 and displays eligible rewards to the member. You can copy and paste the sample code below to your webpage and adapt it as necessary.
//----
//On success of the get_zrl_rewards() call for the user above, display the
//rewards to the user below on the shopping cart page.
show_rewards = function() {
for (i = 0; i < zrl_mi.user_rewards.length; i++) {
//for each eligible reward, process the reward
reward = zrl_mi.user_rewards[i];
if (reward.allowed_redeem_points == 0) {
//If it is a Zero point reward, apply it to the cart directly
//because the customer is eligible for those rewards without any
//any points deduction.
//You will have to write this piece of code
} else {
//Display the available rewards in your webpage for the user to select
//Use the fields described below for each reward
//You will have to write this piece of code
//----Field descriptions----
//reward.redemption_type - Reward Type
//(Percentage Discount / Fixed Amount Discount / Free Shipping)
//reward.giftcard_description - Reward Description
//reward.redemption_name - Reward display name
//reward.allowed_redeem_points - Points deducted for the Reward
//reward.redemption_value - Reward Value in currency e.g. 5 dollars
//reward.redemption_id - Reward ID
//---------------------------
}
}
}
//----
To reiterate, if any of the rewards are available for 0 points, they are not displayed to the member and instead directly applied to the cart.
For example, if the member is eligible for a free shipping reward for 0 points for being a gold tier member, the free shipping reward should be automatically applied. From the remaining rewards, the member should choose which reward he would like to apply to the cart.
Note: Rewards should be displayed to the member only if the member has any eligible rewards. There is no point of showing an empty drop-down list.
Error Function
The code for the error function handle_error used in Step 1 is described here. The error function will need to be written by you.
//----
handle_error = function(){
//handle the error is the Ajax function is not successful
//Best course of action is to choose not to display any eligible rewards
//Or you can retry the Ajax call
//You will need to write this piece of code
}
//----
Step 3: Apply the Reward to the Cart
When the member chooses a reward and clicks on the 'Apply' button, the reward should be applied to the cart. This will be a part of the code you write in the show_rewards function defined in Step 2.
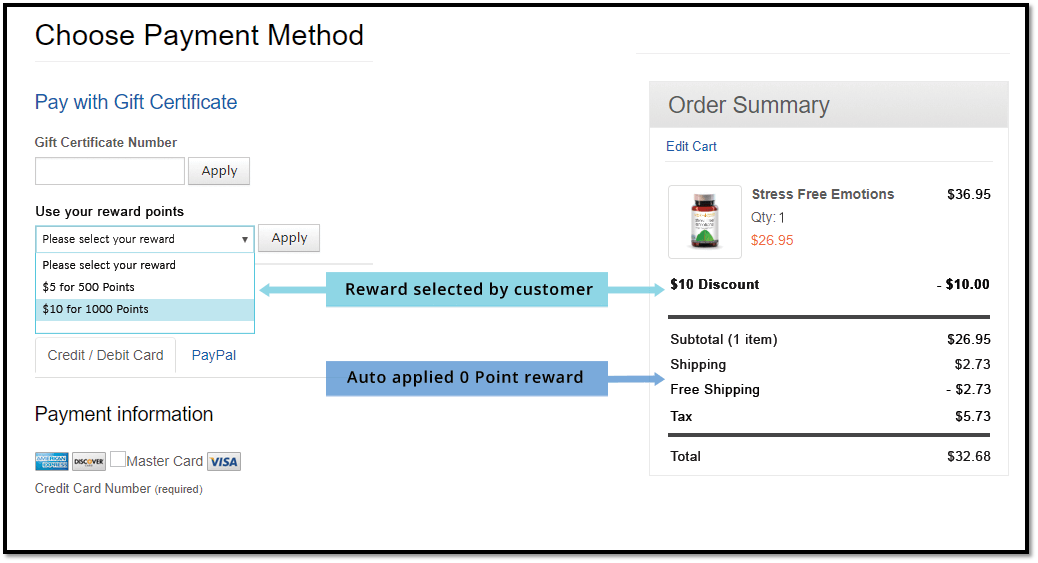
Step 4: Confirm the Redemption
Once the purchase is completed and you are ready to deduct points from the member's loyalty rewards account, call the server-side Redeem API call to deduct the points.
When the ‘order submit’ button is clicked to submit the order, make a separate server- side API call to Zinrelo to confirm each reward that was redeemed. If two loyalty reward redemptions were made, for example, $10 Discount and Free Shipping, then you will need to make two separate Redeem API calls on the server side.
Sample python script to call Zinrelo's Redeem API:
import requests
import json
headers = {'partner-id': 'cad458dc4e',
'api-key': 'c921e097e6679d21c0cad26a45bfec20'}
payload = {"user_email": "[email protected]",
"redemption_id": "reward_16383"}
response = requests.post(url = "https://api.zinrelo.com/v1/loyalty/redeem",
headers = headers, data = payload)
In the JSON response, Zinrelo will pass a “coupon_code” value. Store this value so that it can be passed back to Zinrelo in the Award Points API.
{
"data":{
"user_email": "[email protected]",
"last_name": "Doe",
"first_name": "John",
"redemption_name": "$10 discount",
"redemption_id" : "reward_16383",
"points": 1000,
"points_status": "redeemed",
"coupon_code": "10OFFMAY2018",
"created_time": "03-May-18 19:20:22"
},
"success":true
}
You can update the redemption status of the member using the Redeem API. For example: If a member added a coupon code and abandoned the cart without using the coupon code, then you can update the "status" as "pending." It will keep the reward in a "pending" state until a member performs any activity. And once the member completes the transaction, the "status" can be updated as "approved" or "rejected" using Reject Pending Transaction API.
Expected Result from Redeem API Call:
When the redemption is confirmed, the redeem transaction should reflect in the member’s account in the Zinrelo Admin Console as a redemption entry.
Error Handling for Redeem API Call:
If Zinrelo responds with a 607 error code – “Insufficient redeemable points”, you will need to do the error handling.
The best course of action is to reload the checkout page without the applied rewards and show a message to the user that he doesn’t have sufficient points to redeem the reward.
Updated about 1 year ago