Setting up Returns Tracking
Set up the returns tracking server side API to deduct points when an order is partially or fully returned.
The loyalty program awards points for purchases. When an order is returned, the points should be deducted from the user's account.
The Returns Tracking API passes the data for each return to Zinrelo. These returns are correlated against an earlier corresponding purchase. This Returns Tracking API enables Zinrelo to deduct points for returned purchases. This API is typically called from the server-side when the return transaction is created.
Returns API
The API call is an HTTP POST request to this URL:
POST https://help.zinrelo.com/reference/return-points
Click here for detailed documentation regarding this API.
API Authentication
All API calls have to be authenticated with your API key and partner ID. The API key and partner ID are automatically generated for you when your Zinrelo account is created. These can be found in your Zinrelo admin console under the General >> Settings section.
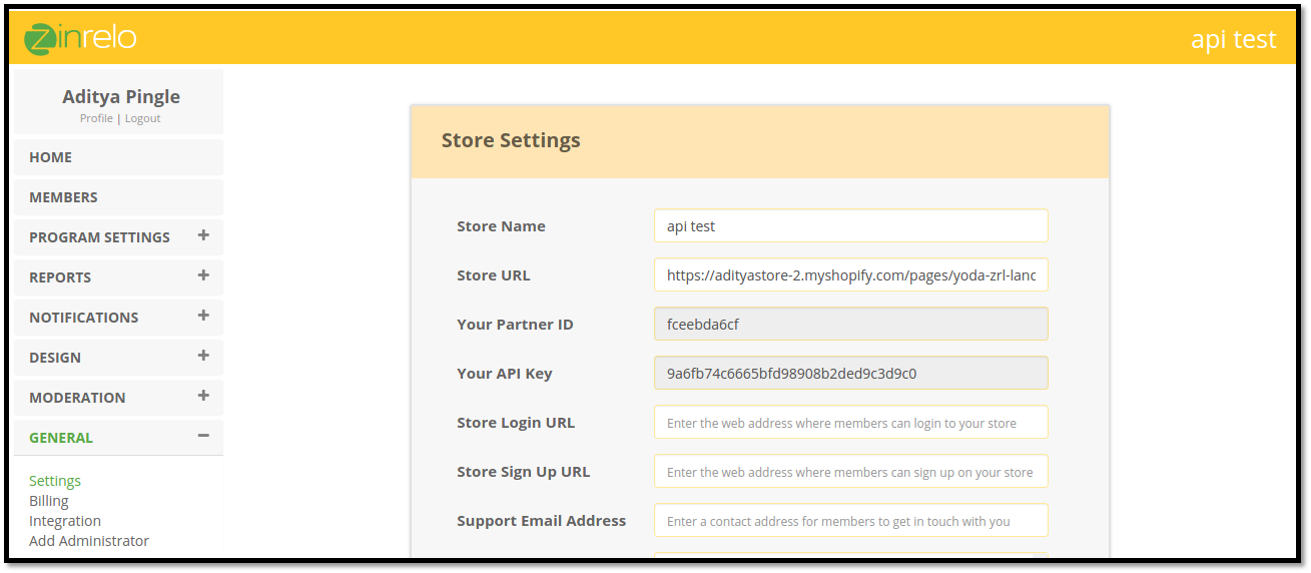
For authenticating an API call, you have to send your Partner ID, and an API Key in the HTTP header of each API request.
Send the keys in the HTTP header of each API request as given below:
'api-key': '<your-api-key'
'partner-id' : '<your-partner-id>'
Calling the Returns API
Shown below is a sample Python script to call the Zinrelo Returns API.
import requests
import json
headers = {'partner-id': 'cad458dc4e',
'api-key': 'c921e097e6679d21c0cad26a45bfec20'}
payload = {
"order_id=75a2726d13artibb10",
"returned_amount = 26.95",
"returned_product_id = 1234df",
"quantity = 1"}
response = requests.post(url = "https://help.zinrelo.com/reference/create-a-return-transaction",
headers = headers, data = payload)
Here is a description of the query parameters used in the payload of the Returns API call.
Parameters | Type | Mandatory | Description |
---|---|---|---|
member_id | string | Yes | Unique Identifier (Member ID) for the member object in the client’s system which was used while creating a member in Zinrelo. |
transaction_attributes | json | Yes | Apart from the above parameters, you have to pass on custom attributes for return activities. Some are pre-defined attributes such as reason and tags, which are common for all activities. In addition to this, you have the ability to define custom attributes against each activity with the parameters like order_id (required field), returned_amount, returned_product_id and returned_product_quantity. |
JSON Response
Here is the sample JSON structured response that you will receive from Zinrelo.
{
"data":{
"user_email": "[email protected]",
"last_name": "John",
"first_name": "Doe",
"points": 270,
"points_status": "pending_deduction",
"returned_for_order_id": "75a2726d13artibb10",
"reason": "Order Fully Returned",
"created_time": "30-Mar-16 19:20:22"
},
"success":true
}
Expected Result
Zinrelo will respond with standard HTTP success or failure codes. In case of a success response, the return transaction will reflect in the customer's account in the Zinrelo admin console.
Error Handling
For failures, Zinrelo will also include extra information about what went wrong, encoded in the response as JSON. The various HTTP and API status codes that Zinrelo returns are listed below.
Standard HTTP status codes:
Code | Title | Description |
---|---|---|
200 | Ok | The request was successful. |
400 | Bad Request | Bad request. |
401 | Unauthorized | Your API key is invalid. |
404 | Not Found | The resource does not exist. |
500 | Internal Server Error | An error occurred with our API. |
503 | Service Unavailable | Service Unavailable. |
Zinrelo API Status Codes:
Status Code | Error Code | Message |
---|---|---|
200 | UNABLE_TO_UPLOAD_PHOTO | Unable to upload the photo. Please try again later. |
400 | ATTRIBUTE_NOT_UNIQUE | IdParams name passed is not a unique attribute. |
200 | MEMBER_ID_ALREADY_IN_USE | Member ID already in use. |
200 | INVALID_MEMBER_STATUS | Invalid member status. |
200 | MEMBER_BLOCKED | Member has been blocked. |
200 | MAX_AWARDS_EXCEEDED | Maximum award limit exceeded. |
200 | INVALID_AMOUNT_TO_DEDUCT | Not enough points to deduct. |
400 | INVALID_JSON | Invalid JSON format. |
400 | INVALID_REQUEST | Invalid request: unsupported Content-Type [content-type]. |
200 | INCORRECT_MEMBER_ID_PASSED | Incorrect Member ID passed. |
200 | STORE_INACTIVE | Store is not active. |
200 | MEMBER_DELETED | Member record has been deleted. |
200 | EXCLUDED_TRANSACTION | Points could not be awarded because exclusion rule was applied. |
404 | TRANSACTION_DOES_NOT_EXIST | Transaction does not exist. |
500 | INTERNAL_SERVER_ERROR | Internal server error. |
400 | INVALID_REQUEST_PARAMETER_VALUES | Invalid request parameter value. |
200 | INVALID_PHOTO | Invalid file type or file size. Please make sure uploaded file type is JPG, JPEG, or PNG. and file size should be less than 10MB. |
200 | PAUSED_ACTIVITY | Activity is in paused state. |
400 | ID_PARAM_NAME_NOT_FOUND | IdParams name passed does not exist. |
401 | ERROR_UNAUTHORIZED | Some error occurred while authenticating your account at Zinrelo. Please verify your API credentials. |
200 | DUPLICATE_ORDER_ID | Loyalty Points already awarded for the Order ID. |
200 | MEMBER_CREATION_UNSUCCESSFUL | Member creation unsuccessful. |
400 | MERCHANT_DOES_NOT_EXIST | Merchant does not exist. |
200 | TRANSACTION_ATTRIBUTE_NOT_UNIQUE | Unique transaction attribute for given activity is not unique. |
200 | INVALID_MEMBER | Member does not exist. |
400 | INVALID_CURSOR | Invalid start cursor provided |
503 | SERVICE_UNAVAILABLE | API Service is unavailable. Please contact Zinrelo support. |
200 | INACTIVE_REWARD | This reward is inactive and cannot be redeemed. |
200 | AUTO_REDEEMABLE_REWARD | Cannot redeem auto redeemable reward via API. |
200 | INVALID_REWARD_FOR_USER | Returned product quantity exceeds order quantity. |
200 | MAX_REWARDS_EXCEEDED | Maximum redemption threshold for reward reached. |
200 | INSUFFICIENT_REDEEMABLE_POINTS | User points balance insufficient. |
200 | INVALID_REWARD_FOR_TIER | This reward is not valid for user tier. |
200 | ORDER_NOT_EXIST | Order with this id does not exist. |
200 | PRODUCT_QUANTITY_EXCEEDED | Returned product quantity exceeds order quantity. |
200 | INVALID_PRODUCT_ID | Incorrect product id passed. |
200 | RETURN_VALUE_EXCEEDS_AVAILABLE_BALANCE | Returned order value exceeds user's points balance. |
200 | PRODUCT_ID_AND_RETURN_AMOUNT_PASSED | Please pass either returned product id or returned amount. |
The error handling code should log the error response. If you need to look into why a return transaction was not processed, the logs will provide that information easily.
Updated about 1 month ago