To authenticate with the UI API, you must generate a JSON Web Token (JWT) and include the partner_id.
To generate the JWT token, you will need an API Key and API key identifier.
Where can I find API key and API key Identifier?
To obtain an API key and API key Identifier, navigate to General >> API keys.
From the list of API keys and identifiers, select the one created for calling Zinrelo endpoints.

To learn how to generate the API key, refer to this help document.
How to generate a JWT token?
You can generate a JWT token using the code snippets provided below in Python, Java, or Ruby:
import jwt
secret = 'your-api-key'
user_info = {
'sub': 'api-key-identifier',
# REQUIRED: Pass API key identifier.
'member_id': 'unique-id',
# REQUIRED: Pass members unique identifier in this field when the user is Logged in.
'exp': 1635862400,
# REQUIRED: Epoch timestamp (seconds), after which the token will expire
}
encoded_jwt = jwt.encode(user_info, secret, algorithm='HS256')
print(encoded_jwt)
import io.jsonwebtoken.*;
import java.util.*;
import org.json.*;
import com.fasterxml.jackson.databind.ObjectMapper;
import java.io.*;
public class JWTApplication {
public static void main(String[] args) throws IOException {
String jwtToken = "";
String secret = "your_api_key"; // New API key
Claims claims = Jwts.claims();
Duration duration = Duration.from(ChronoUnit.HOURS.getDuration());
long epoch = System.currentTimeMillis() / 1000;
long validityInSeconds = 3600;
long exp = epoch + validityInSeconds;
String userInfo = "{\"member_id\" : \"Unique-ID\", \"sub\" : \"API- key-Identifier\"}"; // Added a semicolon here
Map<String, Object> mapping = new ObjectMapper().readValue(userInfo, HashMap.class);
for (String key : mapping.keySet()) {
claims.put(key, mapping.get(key));
}
claims.put("exp", exp);
jwtToken = Jwts.builder().setClaims(claims)
.signWith(SignatureAlgorithm.HS256, Base64.getEncoder().encodeToString(secret.getBytes()))
.compact();
System.out.println(jwtToken);
}
}
require 'jwt'
secret = 'your-api-key' // New API key
user_info = {
'sub' => 'API- key-Identifier',
'member_id' => 'Unique-ID',
'exp' => 1635862400, # REQUIRED: Epoch timestamp (seconds), after which the token will expire
}
encoded_jwt = JWT.encode(user_info, secret, 'HS256')
puts encoded_jwt
Note: If you are using the default API key for the JWT token, there is no need to pass the 'sub' parameter.
Where can I find the partner_id?
- Login to the admin console.
- Navigate to General >> Settings, to find your Partner ID.

Pass JWT and Partner ID in the API
Once you have obtained the JWT and partner ID, you need to include this data when making API requests.
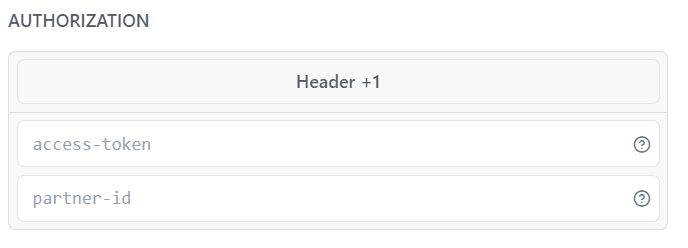